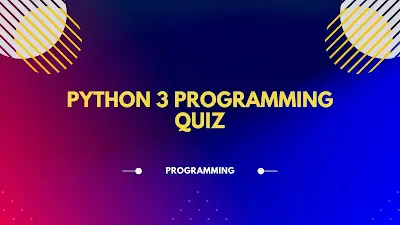
Python 3 Programming Quiz Question Answers
bytearray provides an mutable sequence, making it modifiable.
- True (ans)
- False
Which is the fastest implementation of Python?
- Jython
- Cpython
- Iron Python
- Pypy (ans)
Which describes bytearrays?
- Without an argument, an array of size 0 is created; contains a sequence of integers 0-255 (ans)
- Bytearrays are immutable objects
When using the Python shell and code block, what triggers the interpreter to begin evaluating a block of code?
- semi colon symbol
- execute command
- colon symbol
- closed parenthesis
- blank line (ans)
Which statement accurately defines the bool class?
- Bool class is subclass of array class
- Boolean first returns False then the True value
- Zero values are considered True always
- Boolean Not returns False if the operand is True (ans)
- Zero values are considered True some times.
Using Pop in list will remove the popped up item.
- True (ans)
- False
Dictionary could be copied to another dictionary using which of the following syntax?
- dict_a = dict_b
- dict_a=dict_b.copy() (ans)
- dict_a = dict_b.clear()
- dict_a = copy(dict_b)
Which statement creates the bytes literal when run?
- bytes_literal = b'Copyright \xc2\xa9' (ans)
- bytes_literal = bytes.encoded.count(0x54)
- bytes_literal = bytes(str_literal,'utf-8') (ans)
- bytes_literal = bytes.str.count(0x54)
Values in bytearray should be integers between ___________.
- 0-512
- 0-63
- 0-1024
- 0-255 (ans)
While using slicing in lists, list[0:2] is equivalent to ______.
- list[:-2]
- list[0:3] -1
- list[-2:0]
- list[:2] (ans)
Which statements prevent the escape sequence interpretation?
- escape'col\tcol2\tcol3\t'
- col1\tcol2\tcol3\t
- r'col\tcol2\tcol3\t' (ans)
- subtext'col\tcol2\tcol3\t'
An empty list could be created with the list() alone.
- True (ans)
- False
What is the output of min('Infinity')?
- y
- I (ans)
- 0
- f
Byte datatype can contain only ______ and ______.
- unicode alone
- decimal & hexadecimal
- ascii & hexadecimal characters (ans)
- ascii & unicode
Which of the following options could be used in the tuple object?
- Reverse, Max (ans)
- Append
- Sorted, Lens, Max
Which methods can be used with list objects?
- Reverse , Decode, Lambda
- Decode , Pop , Clear
- Lambda , Pop , Clear
- Reverse , Pop , Clear (ans)
- Reverse , Decode
_______ class provides an immutable sequence of elements.
- tuple (ans)
- bytearray
- string
- list
- byte
Consider b is frozen set, what happens to b.add(3)?
- 3 will be added as a constant
- Error as frozen sets cannot be modified (ans)
- 3 will not be available for other general operations
What characteristics describe the python programming language ?
- Interpreted and open source (ans)
- Interpreted and compiled
- Assembly compiled
Which of the following attributes shows the characteristics of Python?
- Broad Standard Library
- Ubiquity (ans)
- Powerful interpreter
- Object Oriented
What is the output of max('Infinity')?
- I
- f
- 0
- y (ans)
- 8
Which statement correctly assigns X as an infinite number?
- x=int('inf')
- x=infinity
- x=float('inf') (ans)
- X=pos_infinity()
The two main versions of Python include _________.
- Python 3.x and 4.x
- Python 3.x and 5.x
- Python 2.x and 3.x (ans)
- Python 3.x and 6.x
The bool class is subset of ______.
- float
- long
- int (ans)
- char
- string
Python is ubiquitous. Is this true or false?
- True (ans)
- False
Python supports automatic garbage collection.
- True (ans)
- False
Code written in Python 3 is backward compatible with Python 2.
- True
- False (ans)
The following options are the salient features of Python except ___________.
- Broad standard library
- Open source
- Limited platform support (ans)
Equivalent operation for function pow(x, y) is __________.
- x ^ y
- x // y
- x ** y (ans)
- x * y
Simple to learn and understand
Which action must be avoided to prevent the previously-defined names from getting overwritten?
- Import selective objects
- Import selectively with aliases
- Using aliases when importing
- Use the wildcard import (ans)
While using Python IDLE, by how many spaces are the code suites indented?
- 5
- 2
- 3
- 4 (ans)
- 1
What command is used to output text from both the Python shell and within a Python module?
- count_print()
- count()
- System.out.println()
- print() (ans)
- OutputStream()
While using 'bool', all zero values are considered as false and non- zero values are considered as true. Is the statement true or false?
- True (ans)
- False
Is x, y = 5, 6 a valid statement?
- True (ans)
- False
What is the output of bool(0)?
- 1
- 0
- Valid
- False (ans)
- True
Which datatype is represented by int in Python 3?
- Short
- Decimal
- Long (ans)
- float
Which of the following will not yield in declaring x as the datatype of float?
- x=5 (ans)
- x=float.fromhex('A')
- x=int(y)
- x=float(10)
a.symmetric_difference(b) highlights the ________.
- a.union(b) - b.intersection(b)
- a - b
- a.intersection(b) - a.union(b)
- a.union(b) - a.intersection(b) (ans)
a.difference(b) highlights the ________.
- a.intersection(b) - a.union(b)
- a.union(b) - a.intersection(b)
- a.union(b) - b.intersection(b)
- a - b (ans)
All of these range types are correct except ______.
- range(20,40,-2)
- range(20,20,2)
- range(20,20,30)
- range(20,40,'-2') (ans)
Empty dictionary is represented as ________.
- ()
- {} (ans)
- ({})
- []
"What is the output of the following code?
for x in range(1,100, 10):
print (x)"
- 2-20-200
- Error
- 1 11 21 31 41 51 61 71 81 91 (ans)
- 2 20 200
What is the output of the following code? count = 0 while count < 2: print (count, " is less than 2") count = count + 2 else: print (count, " is not less than 2")
- 0 is less than 2; 2 is not less than 2 (ans)
- 1 is less than 2; 3 is not less than 2
- 2 is not less than 2
- Error
"What is the output of the following code? a = 0 if a: print(""""a's value"""") else: print(""""Sorry nothing will get printed"""")"
- Sorry nothing will get printed (ans)
- 0
- Error
- a's value
What is the output of the following code snippet?
for char in 'Welcome':
print (char, end='*')
print()
- Welcome*******
- Error
- W*e*l*c*o*m*e* (ans)
- Welcome*
Which of the given Desktop applications is created using Python?
- Blender
- Web Scripting
- Sametime
- Dropbox
The default decode option available in the byte data type is ________.
- utf-8 (ans)
- ascii
- utf-16
- unicode
list[:] output would be printing the __________.
- Complete list minus first element
- Complete list minus last element
- Complete list (ans)
What is the output of the given code?
list_pt = list('Welcome')
print(list_pt)
['Welcome']\n
- Welcome
- ['W', 'e', 'l', 'c', 'o', 'm', 'e'] (ans)
- ['W', 'e', 'l', 'c', 'o', 'm', 'e']\n
- ['Welcome']
What is the output for the given code?
a = -10
if a:
print("a's value")
else:
print("Sorry nothing will get printed")
- -10
- a's value (ans)
- Sorry nothing will get printed
- Error
All of these range types are correct except ___.
- range(10,20,30)
- range(20,40,-1.2) (ans)
- range(10,20,2)
Which statements will result in slice of tuple?
- a_tuple[:] a_tuple[::-1]
- a_tuple[::2] a_tuple[:]
- a_tuple[::-1] a_tuple[0] =[5]
- a_tuple[0] =[5] a_tuple[::2]
What is the output of the following code?
for x in (2,20,200)
print (x)"
- "2 20 200"
- 220200
- 2 20 200
- error (ans)
In what format does the input function read the user input?
- Object
- Boolean
- Integer
- String (ans)
- Array
- True
- False (ans)
- A list of None's (ans)
- A list of Trues
- A list of Falses
- None of the options
- None (ans)
- Return value must be specified explicitly
- 1
- -1
- [6,4,1]
- [6]
- [1, 4,6,4,1]
- [1,4] (ans)
- True
- False (ans)
- True (ans)
- False
- Variable number of arguments
- Default arguments
- Keyword arguments can be passed after non-keyword arguments
- Required arguments
- All the options (ans)
- Parameter list
- Function name
- Function name and parameter list (ans)
- Return value must be specified explicitly
- float
- dict
- int (ans)
- bool
- ['A', 'B', 'C'] (ans)
- ['a', 'b', 'c']
- ('A', 'B', 'C')
- ('a', 'b', 'c')
- Prints all characters that are not vowels (ans)
- Prints nothing
- Prints all vowels
- Prints all characters
- {97, 112, 108, 101} (ans)
- {'a':97, 'p':112, 'p':112, 'l':108, 'e':101}
- [97, 112, 112, 108, 101]
- {97, 112, 112, 108, 101}
- {0, 1}
- {0, 1, 0, 1, 0, 1, 0, 1} (ans)
- [0, 1, 0, 1, 0, 1, 0, 1]
- [0,1]
- import sys; sys.builtin_module_names (ans)
- import builtins; builtins.builtins_names
- import builtins; builtins.module_names
- import sys; sys.builtins_names
- from m1 import *
- import m1
- from m1 import f1, f2
- import f1, f2 from m1 (ans)
- True (ans)
- False
- optparse
- getopt
- argparse
- cmdparse (ans)
- sample
- pick
- choice (ans)
- choose
- import builtins; builtins.builtins_names
- import sys; sys.builtins_names
- import sys; sys.builtins.dict.keys()
- import builtins; builtins.dict.keys() (ans)
- os.isFile(C:\Sample.txt)
- os.path.isFile(C:\Sample.txt)
- os.path.exists(C:\Sample.txt)
- os.path.isfile(C:\Sample.txt) (ans)
- repmanage
- pkgmanage
- pip (ans)
- zip
- init.py
- main.py
- init.py (ans)
- main.py
- tarfile
- zlib
- lzma
- All of those mentioned (ans)
- NonObjectError
- NameError
- VariableError (ans)
- UndefinedError
- NonDivisibleError
- ArithmeticError
- ZeroDivisionError (ans)
- DivisionError
- When an exception occurs in to except block
- When an exception occurs
- When no exception occurs (ans)
- Always
- Zero (ans)
- More than one
- One
- More than zero
- ArithemticError
- NameError
- ValueError
- TypeError
- Only when there is an exception
- Always (ans)
- Only when there is no exception
- Never